Recursion Prints Over and Over Again
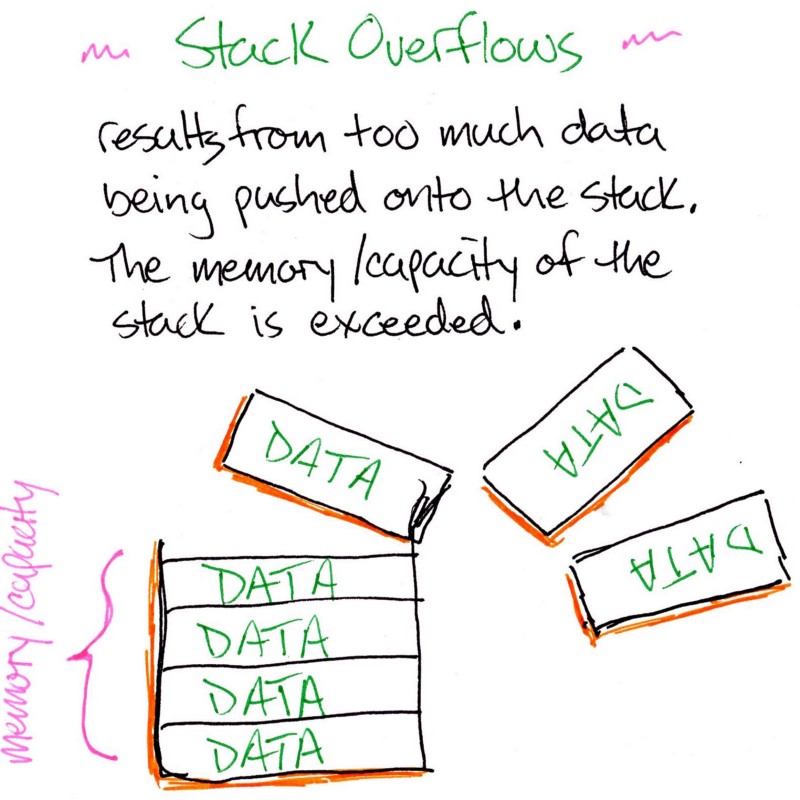
by Kevin Turney
Recursion is not hard: a step-by-step walkthrough of this useful programming technique
I'one thousand going to say this right off the bat. Practice you know the events that happen upon function invocation? No? And then that'south where we will showtime.
Function invocation
When we telephone call a role, an execution context gets placed on the execution stack. Let's break this downwardly some more than.
First, what is a stack?
A stack is a data structure that operates on a "Last In, First Out" basis. An item is "pushed" onto a stack to add to it, and an item is "popped" off the stack to remove it.
Using a stack is a method of ordering certain operations for execution.
Now, back to what is an execution context? An execution context forms upon a office invocation. This context places itself on an execution stack, an order of operations. The item that is always first in this stack is the global execution context. Next up are any function created contexts.
These execution contexts accept properties, an Activation Object and a "this" binding. The "this" binding is a reference dorsum to this execution context. The Activation Object includes: parameters passed, declared variables, and role declarations.
So every fourth dimension nosotros place a new context on the stack, we usually have everything we need to execute lawmaking.
Why do I say ordinarily?
With recursion, we are waiting for return values coming from other execution contexts. These other contexts are college upward the stack. When the concluding particular on the stack finishes execution, that context generates a render value. This return value gets passed down as a render value from the recursive example to the next detail. That execution context is then popped off the stack.
Recursion
So, what is recursion?
A recursive office is a function that calls itself until a "base of operations condition" is true, and execution stops.
While false, nosotros volition go along placing execution contexts on height of the stack. This may happen until we take a "stack overflow". A stack overflow is when we run out of memory to agree items in the stack.
In general, a recursive function has at least two parts: a base condition and at least one recursive case.
Let'south wait at a classic instance.
Factorial
const factorial = function(num) { debugger; if (num === 0 || num === 1) { return ane } else { return num * factorial(num - one) }}
factorial(5)
Here nosotros are trying to find 5! (v factorial). The factorial function is divers as the product of all positive integers less than or equal to its argument.
The start condition states: "if the parameter passed equals 0 or 1, we will exit and render 1".
Side by side, the recursive example states:
"If the parameter is non 0 or i, and so nosotros will pass value of num
times the return value of calling this role again with num-ane
as its statement".
And so if we call factorial(0)
, the function returns ane and never hits the recursive example.
The same holds for factorial(one)
.
We can encounter what is happening if we insert a debugger statement into the code and utilize devtools to step though it and watch the call stack.
- The execution stack places
factorial()
with v as the argument passed. The base instance is false, then enter the recursive condition. - The execution stack places
factorial()
a 2d time withnum-1
= 4 equally argument. Base case is false, enter recursive status. - The execution stack places
factorial()
a tertiary time withnum-1
(4–i) = three every bit statement. Base of operations example is false, enter recursive condition. - The execution stack places
factorial()
a fourth time withnum-ane
(iii–1) = 2 as argument. Base example is false, enter recursive status. - The execution stack places
factorial()
a 5th time withnum-1
(two–1) = 1 as argument. Now the base of operations case is truthful, so return ane.
At this indicate, we have decreased the argument by i on each function call until nosotros achieve a condition to return 1.
6. From here the last execution context completes, num === 1
, and so that function returns 1.
7. Side by side num === ii
, so the return value is 2. (i×2).
viii. Next num === 3
, sothe return value is vi, (2×3).
So far we have 1×2×iii.
9. Next, num === 4
, (4×vi). 24 is the return value to the side by side context.
10. Finally, num === v
, (5×24) and we have 120 every bit the final value.
Recursion is pretty smashing, right?
Nosotros could have done the same thing with a for or a while loop. But using recursion yields an elegant solution that is more than readable.
This is why we use recursive solutions.
Many times, a problem broken downwardly into smaller parts is more efficient. Dividing a problem into smaller parts aids in conquering it. Hence, recursion is a divide-and-conquer approach to solving problems.
- Sub-bug are easier to solve than the original trouble
- Solutions to sub-problems are combined to solve the original problem
"Split-and-conquer" is nigh often used to traverse or search information structures such as binary search trees, graphs, and heaps. It as well works for many sorting algorithms, like quicksort and heapsort.
Let's work through the post-obit examples. Use devtools to get a conceptual grasp of what's happening where and when. Call up to use debugger statements and step though each procedure.
Fibonacci
const fibonacci = function(num) { if (num <= 1) { return num } else { render fibonacci(num - one) + fibonacci(num - 2) }}fibonacci(5);
Recursive arrays
part flatten(arr) { var result = [] arr.forEach(role(element) { if (!Array.isArray(element)) { result.button(element) } else { consequence = result.concat(flatten(element)) } }) render result}
flatten([1, [two], [three, [[4]]]]);
Reversing a string
function reverse(str) { if (str.length === 0) render '' render str[str.length - 1] + opposite(str.substr(0, str.length - 1))}
contrary('abcdefg');
Quicksort
function quickSort(arr, lo, hello) { if (lo === undefined) lo = 0 if (hi === undefined) how-do-you-do = arr.length - 1
if (lo < hi) { // partition the assortment var p = sectionalization(arr, lo, hi) console.log('partition from, ' + lo + ' to ' + hi + '=> partition: ' + p) // sort subarrays quickSort(arr, lo, p - 1) quickSort(arr, p + 1, hi) } // for initial call, render a sorted assortment if (hi - lo === arr.length - 1) return arr}
function sectionalization(arr, lo, hi) { // choose concluding element as pivot var pin = arr[hi] // keep track of index to put pivot at var pivotLocation = lo // loop through subarray and if chemical element <= pin, identify chemical element earlier pivot for (var i = lo; i < hi; i++) { if (arr[i] <= pin) { swap(arr, pivotLocation, i) pivotLocation++ } } swap(arr, pivotLocation, hello) return pivotLocation}
function swap(arr, index1, index2) { if (index1 === index2) render var temp = arr[index1] arr[index1] = arr[index2] arr[index2] = temp console.log('swapped' + arr[index1], arr[index2], +' in ', arr) return arr}
quickSort([1, 4, 3, 56, 9, eight, seven, five])
Practicing recursive techniques is important. For nested data structures similar copse, graphs, and heaps, recursion is invaluable.
In a futurity article, I will hash out tail-call optimization and memoization techniques as they relate to recursion. Thanks for reading!
Further resources
Wikipedia
Software Engineering
Another proficient article
M.I.T. open courseware
Learn to lawmaking for gratuitous. freeCodeCamp's open up source curriculum has helped more 40,000 people get jobs as developers. Get started
jenningswhintaked.blogspot.com
Source: https://www.freecodecamp.org/news/recursion-is-not-hard-858a48830d83/
0 Response to "Recursion Prints Over and Over Again"
Post a Comment